How to Create Your Own Custom Shortcode in WordPress
Shortcodes can be really useful when you want to add dynamic content or custom code inside the WordPress post and pages. However, if you want to create a custom shortcode, then it requires some coding experience.
If you are comfortable with writing PHP code, then here is a sample code that you can use as a template.
// function that runs when shortcode is called
function ads_shortcode() {
// Things that you want to do.
$message = 'Hello world!';
// Output needs to be return
return $message;
}
// register shortcode
add_shortcode('my-ads-code', 'ads_shortcode');
In this code, we first created a function that runs some code and returns the output. After that, we created a new shortcode called ‘my-ads-code’ and told WordPress to run the function we created.
You can now use add this shortcode to your posts, pages, and widgets using the following code: [my-ads-code]. It will run the function you created and show the desired output.
For example, i’ll create a shortcode to show Google Ads by edit Theme Functions (functions.php).
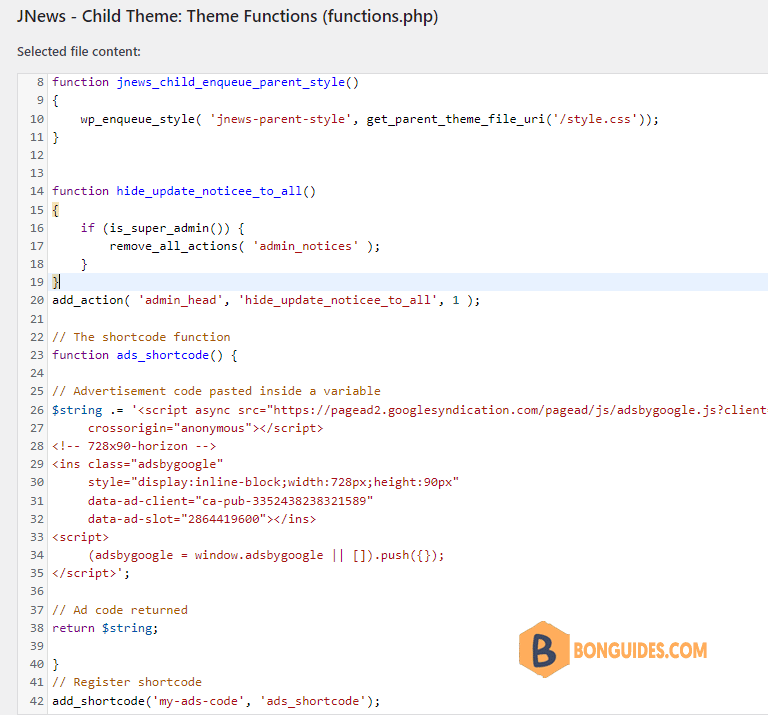
// The shortcode function
function ads_shortcode() {
// Advertisement code pasted inside a variable
$string .= '<script async src="https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js?client=ca-pub-3352438238321589"
crossorigin="anonymous"></script>
<!-- 728x90-horizon -->
<ins class="adsbygoogle"
style="display:inline-block;width:728px;height:90px"
data-ad-client="ca-pub-3352438238321589"
data-ad-slot="2864419600"></ins>
<script>
(adsbygoogle = window.adsbygoogle || []).push({});
</script>';
// Ad code returned
return $string;
}
// Register shortcode
add_shortcode('my-ads-code', 'ads_shortcode');
Don’t forget to replace the ad code with your own advertisement code. You can now use the [my-ads-code] shortcode inside your WordPress posts, pages, and sidebar widgets.
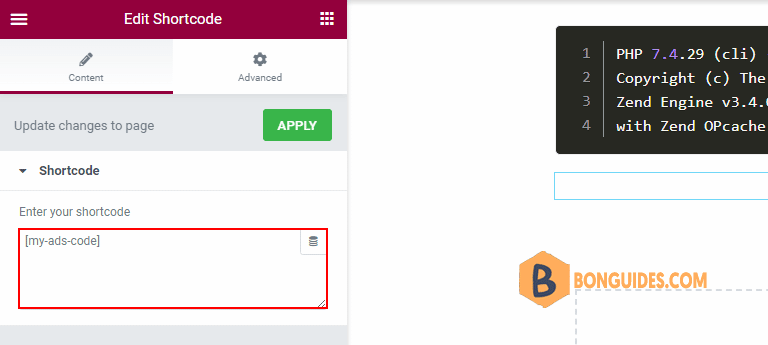
WordPress will automatically run the function associated with the shortcode and display the advertisement code.
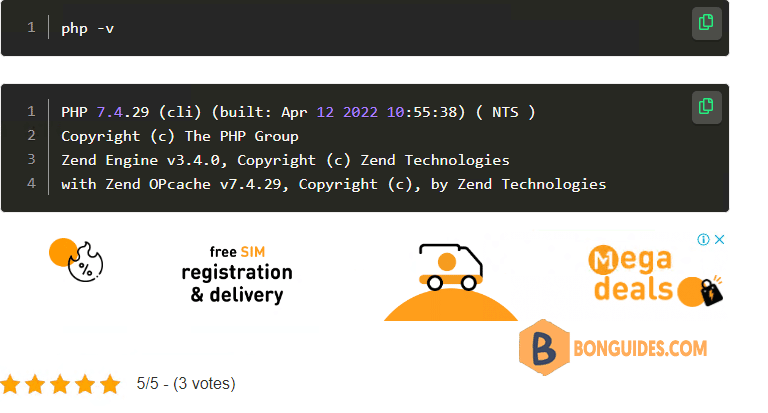
How to Add a Shortcode in WordPress Theme Files
Shortcodes are meant to be used inside WordPress posts, pages, and widgets. However, sometimes you may want to use a shortcode inside a WordPress theme file.
Basically, you can add a shortcode to any WordPress theme template by simply adding the following code.
<?php echo do_shortcode("[your_shortcode]"); ?>
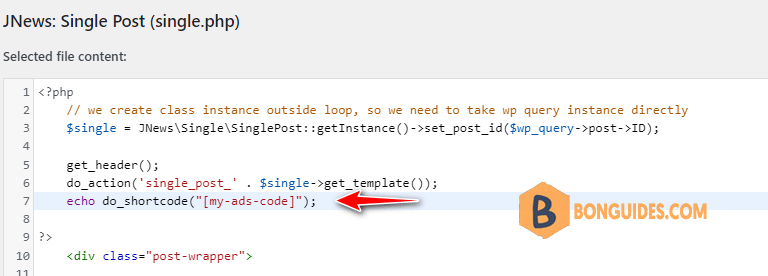