Table of Contents
This article will show you how to get the creation date of all your Azure Active Directory users using a PowerShell script. This is important for auditing purposes and can be used in other scripts to remove any users that were created after a certain date.
Export Creation Date Entra ID Users
1️⃣ First, you must install Entra ID PowerShell module and Connect to Entra ID PowerShell.
Optional: If you don’t have it, let’s open Windows PowerShell as administrator then run the following cmdlets:
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope LocalMachine -Force
Install-PackageProvider -Name NuGet -Force
Set-PSRepository -Name "PSGallery" -InstallationPolicy Trusted
Install-Module -Name AzureAD
Import-Module AzureAD
Connect-AzureAD
2️⃣ Run the following script to export the creation date of all users in your Azure Active Directory.
$DataArray = @()
$ADUsers = get-azureaduser -all:$true
foreach($User in $ADUsers) {
$Creation = Get-AzureADUserExtension -objectid $user.UserPrincipalName
$UserUPN = $user.UserPrincipalName
$UserDisplayName = $User.DisplayName
$TableRow = "" | Select DisplayName, UserPrincipalName, CreationDate
$TableRow.DisplayName = $UserDisplayName
$TableRow.UserPrincipalName = $UserUPN
$TableRow.CreationDate = $Creation.createdDateTime
$DataArray += $TableRow
}
$DataArray | format-table
$DataArray | format-table
DisplayName UserPrincipalName CreationDate
----------- ----------------- ------------
Bon Ben [email protected] 7/3/2022 2:39:01 AM
Anna [email protected] 7/4/2022 2:55:52 PM
Chris [email protected] 7/3/2022 2:32:20 PM
David [email protected] 7/19/2022 2:22:18 PM
Maria [email protected] 7/18/2022 3:49:54 AM
May [email protected] 7/19/2022 9:20:53 AM
mg [email protected] 8/8/2022 6:49:31 AM
Tom [email protected] 7/4/2022 2:57:47 PM
Tommy Luk [email protected] 7/27/2022 9:01:04 AM
Tonny [email protected] 7/11/2022 1:41:20 PM
If you want to export the result to a CSV file, let’s run the following command.
$DataArray | Export-Csv C:\Report_CreationDate.csv -Nti
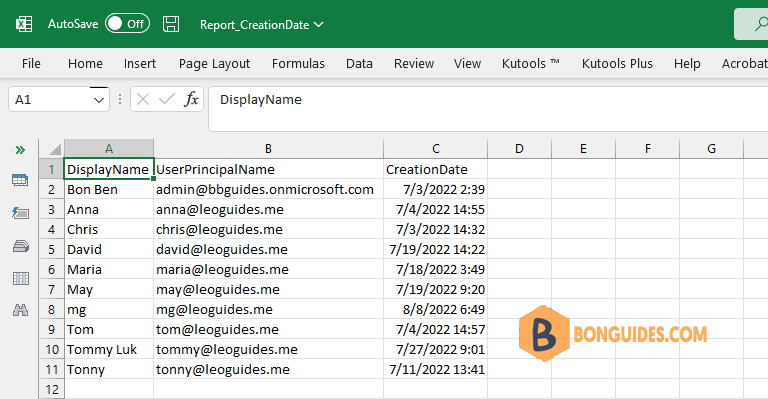
Get the creation date for a single user
In some cases, you just need the creation date of a single users instead of all users in your organization. Let’s using the following command.
(Get-AzureADUserExtension -objectid [email protected]).createdDateTime
Script using PSCustomObbject
$Report = @()
$Users = Get-AzureADUser -All:$true
foreach ($User in $Users)
{
$ObjReport = [PSCustomObject]@{
DisplayName = $User.DisplayName
UserPrincipalName = $User.UserPrincipalName
CreationDate = (Get-AzureADUserExtension -ObjectId $User.ObjectId).Get_Item("createdDateTime")
}
$Report += $ObjReport
}
$Report | Format-Table