Table of Contents
Download the Latest Package from GitHub
Lately I’ve been working on an application for which the releases are published on GitHub, and I wanted to use PowerShell to always downloads the latest package release.
It turned out that downloading the artifacts for a specific version is easy, we can simply build an URL with the version number to access the artifact. However, there is no direct URL to download artifacts from the latest release, so we need a bit of logic in PowerShell to do that.
Link structure
We can access a particular release with a URL like https://github.com/<user>/<repo>/releases/tag/v1.x.x. (The part after tag/ is what we specified when we created the release.)

Artifacts from this particular release can be downloaded with the URL https://github.com/<user>/<repo>/releases/download/v1.x.x/myArtifact.zip

Getting the latest version from GitHub
Take a look the link of zlib project https://github.com///releases/latest.
- <user> = madler
- <repo> = zlib
The following example demonstrates this by fetching the latest Windows x64 release .zip file of the PowerShell: https://github.com/PowerShell/PowerShell/releases/latest

$url = 'https://github.com/PowerShell/PowerShell/releases/latest'
$request = [System.Net.WebRequest]::Create($url)
$response = $request.GetResponse()
$tagUrl = $response.ResponseUri.OriginalString
$version = $tagUrl.split('/')[-1].Trim('v')
$fileName = "PowerShell-$version-win-x64.zip"
###Create the download link
$downloadUrl = $tagUrl.Replace('tag', 'download') + '/' + $fileName
###Create a folder then download to the temp folder
New-Item -Path $env:TEMP/pwsh -ItemType Directory -Force
Invoke-WebRequest -Uri $downloadUrl -OutFile $env:TEMP/pwsh/$fileName
###Open the destination folder
Invoke-Item $env:TEMP/pwsh
Downloading the latest version of PowerShell from GitHub using PowerShell.
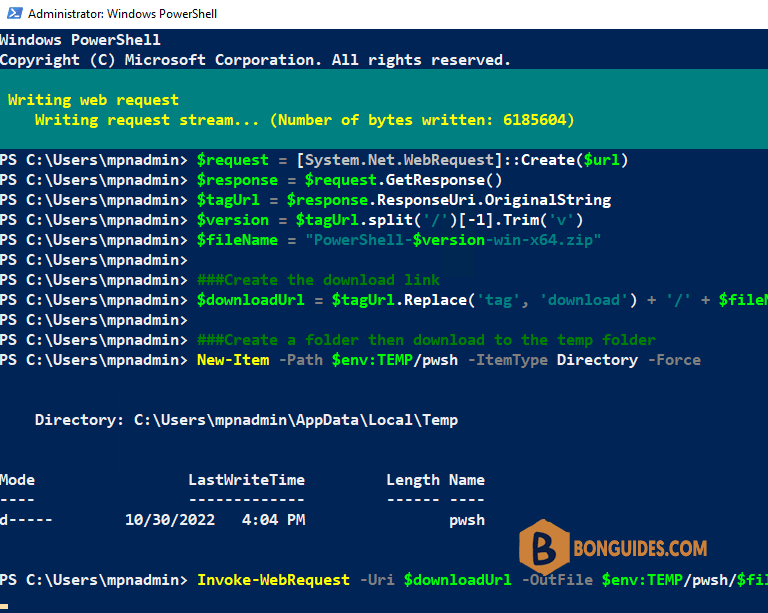
Once done, you can see the package is saved in the destination folder.
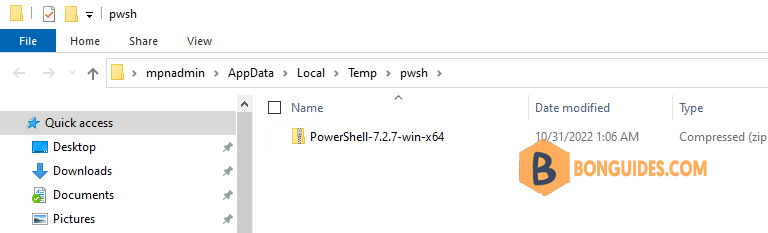